EAN-13 Barcode Check Digit Calculator
Càpita di dover controllare al volo la validità di un codice a barre EAN13 e quindi il relativo check digit.
Ecco una semplice utility in MFC che esegue il check del carattere di controllo.
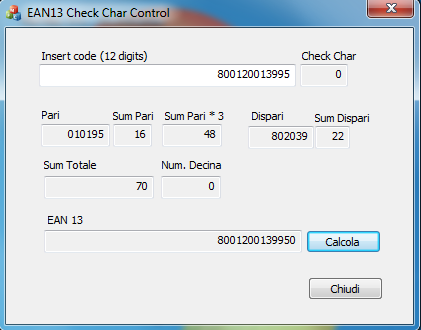
Note: Installare Microsoft Visual C++ 2010 Redistributable Package (x86);
Visual C++:
#include "stdafx.h" #include "BCodeCheckTest.h" #ifdef _DEBUG #define new DEBUG_NEW #endif // L'unico oggetto applicazione CWinApp theApp; using namespace std; typedef wchar_t *(__stdcall *CHECK_NUM)(wchar_t *_ean); int _tmain(int argc, TCHAR* argv[], TCHAR* envp[]) { //Inizializzazione MFC int nRetCode = 0; HMODULE hModule = ::GetModuleHandle(NULL); if (hModule != NULL) { // inizializza MFC e visualizza un messaggio in caso di errore if (!AfxWinInit(hModule, NULL, ::GetCommandLine(), 0)) { // TODO: modificare il codice di errore in base alle esigenze _tprintf(_T("Errore irreversibile: inizializzazione di MFC non riuscita\n")); nRetCode = 1; } else { // TODO: codificare il comportamento dell'applicazione. HMODULE hMd = LoadLibrary(_T("bcodesupport_x86.dll")); if(hMd != NULL) { //Chiamo la funzione GetCheckNum nella DLL wchar_t *m_ean12_chars = _T("800317001479"); CHECK_NUM CheckNumber; CheckNumber = (CHECK_NUM) GetProcAddress(hMd,(LPCSTR)("GetCheckNum")); wchar_t *m_CheckChar = CheckNumber(m_ean12_chars); wcout << "Check digit : " << (LPCSTR)(m_CheckChar) << endl << endl; wcout << "EAN13 : " << m_ean12_chars << (LPCSTR)(m_CheckChar) << endl << endl << "Premere un tasto per continuare..." << endl; getchar(); } FreeLibrary(hMd); } } else { // TODO: modificare il codice di errore in base alle esigenze. _tprintf(_T("Errore irreversibile: GetModuleHandle non riuscito\n")); nRetCode = 1; } return nRetCode; }
using System; using System.Collections.Generic; using System.ComponentModel; using System.Data; using System.Drawing; using System.Linq; using System.Text; using System.Windows.Forms; using System.Runtime.InteropServices; namespace bcodecheck { public partial class Form1 : Form { //Compilare settando : "Piattaforma di destinazione x86" //se usi la versione x86; //"Any CPU" se usi la versione x64; [DllImport("bcodesupport_x86.dll", CallingConvention=CallingConvention.StdCall, CharSet=CharSet.Unicode)] public static extern IntPtr GetCheckNum(string bcode); public Form1() { InitializeComponent(); } private void button1_Click(object sender, EventArgs e) { string val = Marshal.PtrToStringAnsi(GetCheckNum(textBox1.Text)); textBox2.Text = val; } } }
unit Unit2; interface uses Winapi.Windows, Winapi.Messages, System.SysUtils, System.Variants, System.Classes, Vcl.Graphics, Vcl.Controls, Vcl.Forms, Vcl.Dialogs, Vcl.StdCtrls; function GetCheckNum(ean:PAnsiChar):PansiChar;stdcall;external 'bcodesupport_x86.dll'; type TForm2 = class(TForm) Button1: TButton; Edit1: TEdit; Edit2: TEdit; Edit3: TEdit; Label1: TLabel; Label2: TLabel; Label3: TLabel; procedure Button1Click(Sender: TObject); private { Private declarations } public { Public declarations } end; var Form2: TForm2; implementation {$R *.dfm} procedure TForm2.Button1Click(Sender: TObject); var ean12 : PAnsiChar; begin ean12 := PAnsiChar(Edit1.Text); Edit2.Text := GetCheckNum(ean12); Edit3.Text := Edit1.Text + Edit2.Text; end; end.